Connect to Deployment Using React Native via MQTT.js SDK
This article mainly introduces how to use MQTT.js in a React Native application built with Expo, and implement the connection, subscription, messaging, unsubscribing and other functions between the client and MQTT broker.
Prerequisites
Deploy MQTT Broker
- You can use the free public MQTT broker provided by EMQX. This service was created based on the EMQX Platform. The information about broker access is as follows:
- Address: broker.emqx.io
- WebSocket Port: 8083
- WebSocket over TLS/SSL Port: 8084
- You can also create your own MQTT broker. After the deployment is in running status, you can find connection information on the deployment overview page. And for the username and password required in the later client connection stage, you can navigate to the Access Control -> Authentication for the setting.
Create a React Native Application
Create new React Native applications with Expo
:
npx create-expo-app@latest mqtt-test
Reference link: https://docs.expo.dev/get-started/create-a-project/.
Install Dependencies
MQTT.js is a fully open-source client-side library for the MQTT protocol, written in JavaScript and available for Node.js and browsers. For more information and usage of MQTT.js
, please refer to the MQTT.js GitHub.
MQTT.js can be installed via npm, yarn or pnpm. This example will install MQTT.js through npm command.
Install via the command line:
shellnpm install mqtt # or yarn add mqtt # or pnpm add mqtt
Import MQTT.js where needed:
jsimport mqtt from "mqtt";
Connect over WebSocket Port
You can set a client ID, username, and password with the following code. The client ID should be unique.
const clientId =
"emqx_react_native_" + Math.random().toString(16).substring(2, 8);
const username = "emqx_test";
const password = "emqx_test";
You can establish a connection between the client and the MQTT broker using the following code:
const client = mqtt.connect("ws://broker.emqx.io:8083/mqtt", {
clientId,
username,
password,
// ...other options
});
Connect over WebSocket Secure Port
If TLS/SSL encryption is enabled, the connection parameter options are the same as for establishing a connection via the WebSocket port, you just need to be careful to change the protocol to wss
and match the correct port number.
You can establish a connection between the client and the MQTT broker using the following code:
const client = mqtt.connect("wss://broker.emqx.io:8084/mqtt", {
clientId,
username,
password,
// ...other options
});
Subscribe and Publish
Subscribe to Topics
Specify a topic and the corresponding QoS level to be subscribed.
const doSubscribe = () => {
setSubBtnText("Subscribing...");
client?.subscribe(subTopic, { qos: 0 }, (error) => {
if (error) {
console.error("Failed to subscribe to topic:", subTopic, error);
setSubBtnText("Subscribe");
} else {
setSubBtnText("Subscribed");
console.log("Subscribed to topic:", subTopic);
}
});
};
Unsubscribe to Topics
You can unsubscribe using the following code, specifying the topic to be unsubscribed.
const doUnsubscribe = () => {
client?.unsubscribe(subTopic, {}, (error) => {
if (error) {
console.error("Failed to unsubscribe from topic:", subTopic, error);
setSubBtnText("Subscribe");
} else {
console.log("Unsubscribed from topic:", subTopic);
setSubBtnText("Subscribe");
}
});
};
Publish Messages
When publishing a message, the MQTT broker must be provided with information about the target topic and message content.
const doPublish = () => {
client?.publish(pubTopic, pubMessage, { qos: 0 }, (error) => {
if (error) {
console.error("Failed to publish message:", error);
} else {
console.log("Message published to topic:", pubTopic);
}
});
};
Receive Messages
The following code listens for message events and save the received message.
client.on("message", (topic, payload) => {
setReceivedMsg((prevMsg) => {
return prevMsg.concat(`\n${payload.toString()}`);
});
});
Disconnect from MQTT Broker
To disconnect the client from the broker, use the following code:
const doDisconnect = () => {
client?.end();
setClient(null);
setConnectBtnText("Connect");
};
The above section only shows some key code snippets, for the full project code, please refer to MQTT Client - React-Native-Expo. You can download and try it out yourself.
Test the Connection
We have written the following simple application using React Native with the ability to create connections, subscribe to topics, send and receive messages, unsubscribe, and disconnect.
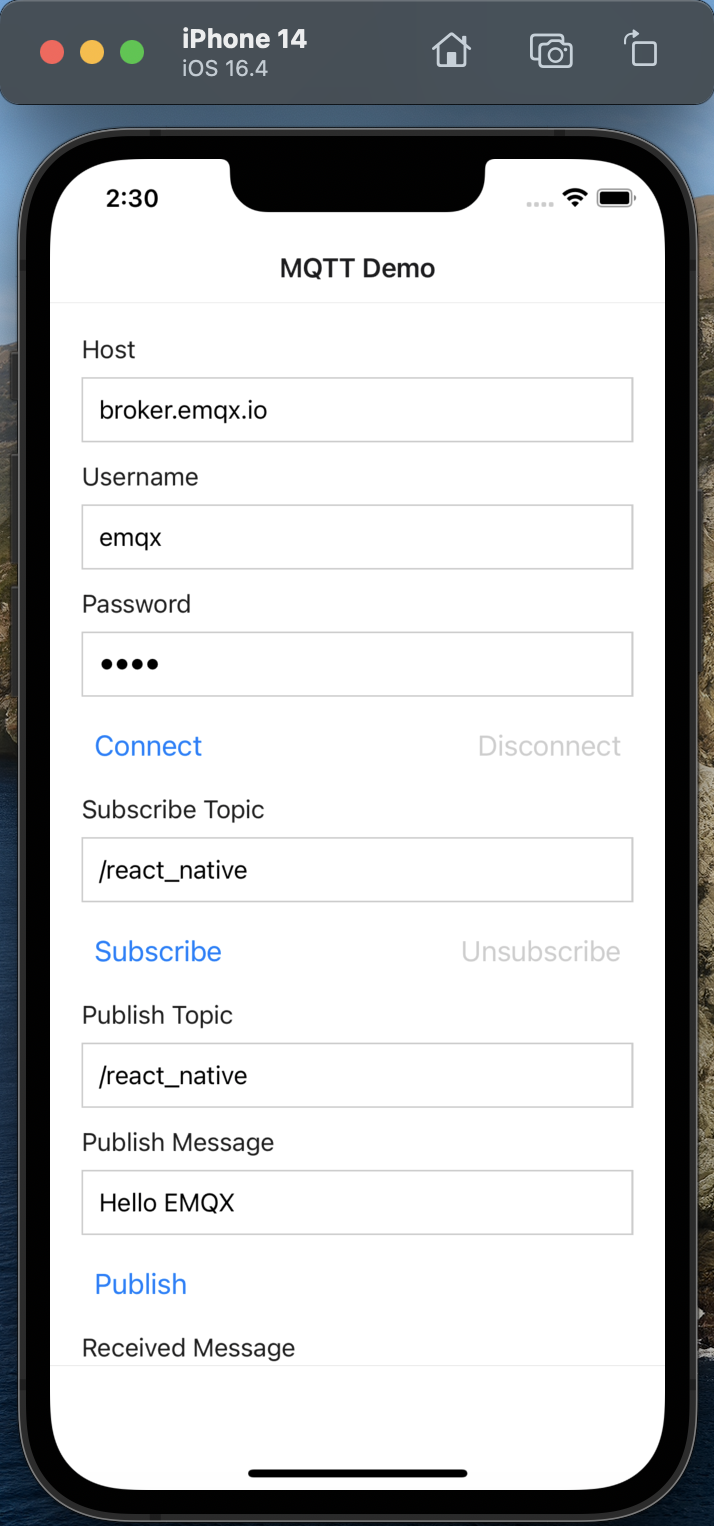
Use MQTT 5.0 client tool - MQTTX as another client to test sending and receiving messages.
You can see that MQTTX can receive messages from the React Native side normally, as can be seen when sending a message to the topic using MQTTX.
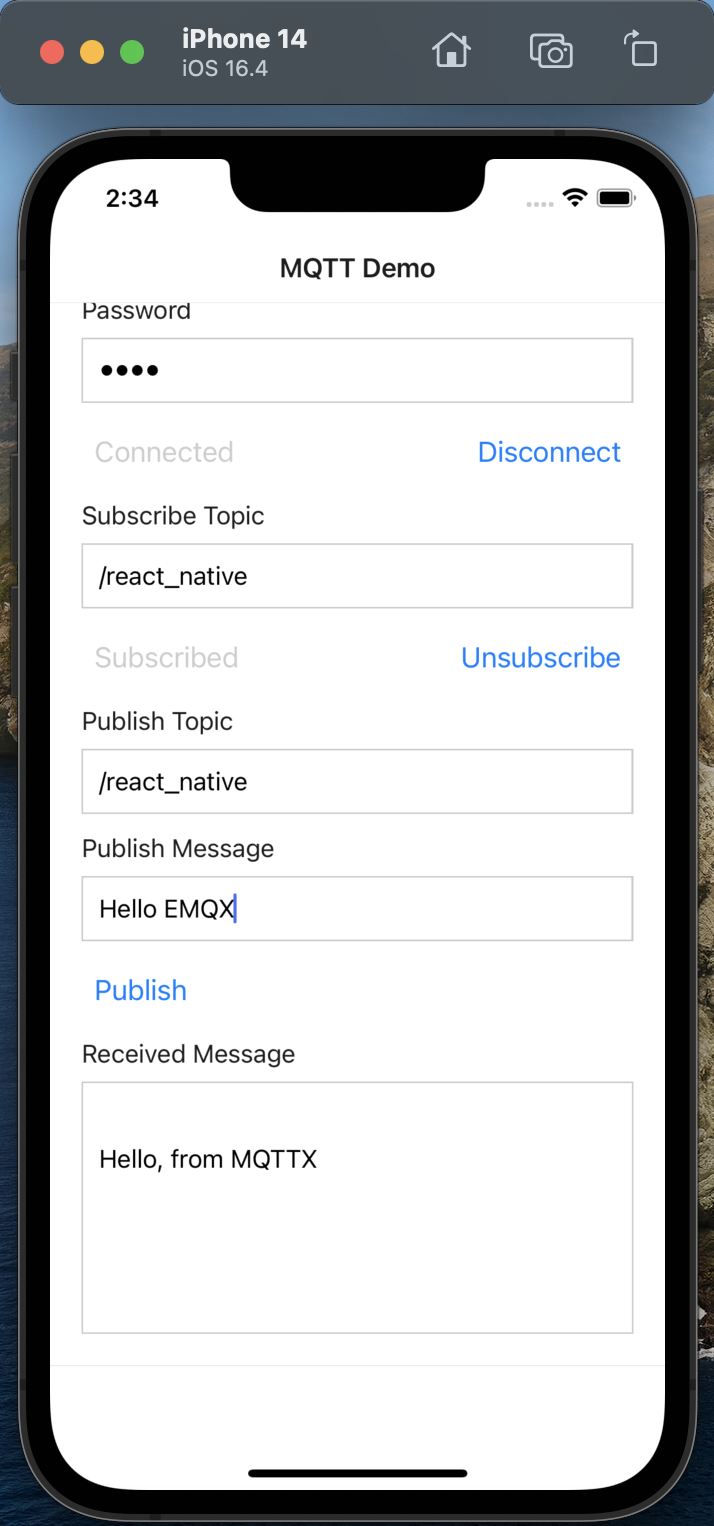
More Information
In conclusion, we have implemented creating MQTT connections in a React Native project and simulated scenarios of subscribing, publishing messages, unsubscribing, and disconnecting between clients and MQTT servers.
You can download the complete example source code on the MQTT Client - React-Native-Expo, and we also welcome you to explore more demo examples in other languages on the MQTT Client example page.